In the world of programming and scripting, managing files securely is crucial. If you’re using Bash for your scripts, you might need to implement file locking to prevent multiple processes from accessing the same file simultaneously. This article will guide you through creating a Bash script that demonstrates how to lock a file effectively.
Prerequisites
- Bash scripting knowledge, including variables and functions.
- Understanding of file permissions and ownership in Unix-like systems.
- A basic text editor, such as
nano
orvim
, for scripting. - No additional packages needed, as this script uses built-in Bash features.
DID YOU KNOW?
File locking is a common technique used to prevent data corruption in multi-process applications.
The Script
This Bash script attempts to create a lock on a specified file. If the lock is successful, it proceeds with operations on the file; otherwise, it informs the user that the file is currently locked.
#!/bin/bash
lock_file="/tmp/mylockfile.lock"
# Attempt to create a lock
exec 200>$lock_file
flock -n 200 || { echo "File is already locked"; exit 1; }
# Critical section starts here
echo "Locked and working on the file..."
# Simulate work with sleep
sleep 5
# Critical section ends here
# Release the lock
rm $lock_file
echo "Lock released."
Step-by-Step Explanation
NOTE!
This script is designed to work on Unix-like systems with Bash and flock installed.
Here’s a detailed breakdown of what the script does:
- Lock File Creation: The script defines the path for the lock file and attempts to open it for writing using file descriptor 200.
- File Locking: It uses
flock -n
to attempt to obtain a lock without waiting. If the lock cannot be obtained, it exits with a message. - Critical Work Section: If the lock is successful, it simulates some work (represented by
sleep
) while holding the lock. - Lock Release: Finally, it removes the lock file, releasing the lock so that other processes can access the file.
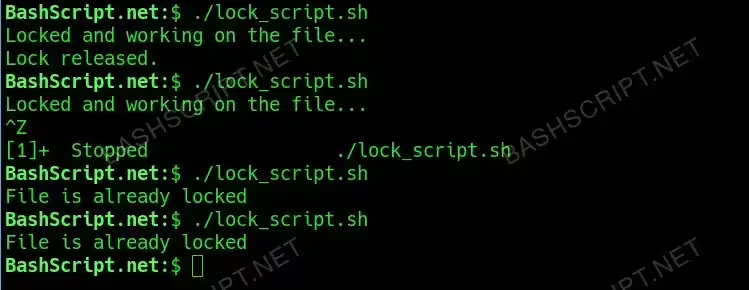
How to Run the Script
Follow these steps to execute the script:
- Open your terminal.
- Create a new file with the script by running
nano lock_script.sh
. - Paste the script into the file and save it.
- Make the script executable with
chmod +x lock_script.sh
. - Finally, run the script using
./lock_script.sh
.
Conclusion
In summary, file locking in Bash can secure your scripts against concurrency issues. By following the steps outlined in this article, you can ensure that your file operations are both safe and efficient.
FAQ
-
What happens if the script is interrupted?
If the script is interrupted, the lock file will not be removed. You may need to manually delete it to regain access.
-
Can I specify a different lock file location?
Yes, modify the
lock_file
variable at the start of the script to point to your desired location. -
Does this work with multiple scripts?
Absolutely! Multiple instances of the script will respect the lock and avoid concurrent access based on the same lock file.
-
Is
flock
available on all systems?Most Unix-like systems include
flock
by default, but you can check its availability usingwhich flock
. -
What error messages might I encounter?
You might see messages regarding permission issues if you try to create the lock file in a restricted directory.
Troubleshooting
Here are some common error messages you might encounter when using the script, along with their solutions:
- Error: “File is already locked” – Ensure no other instance is running or delete the lock file manually.
- Error: “Permission denied” – Check your permissions for the directory where the lock file is being created.
- Error: “No such file or directory” – Ensure the script file exists and is called correctly.