Managing concurrent processes in a Bash script can be a challenging endeavor, especially when tasks need to be executed without overlap. This is where file locking comes into play. In this article, we will explore how to use the flock command in Bash scripts to create file locks, ensuring that only one instance of a task can run at a time. This capability prevents unexpected behaviors and resource contention, making our scripts safer and more reliable.
Prerequisites
DID YOU KNOW?
Using file locks can significantly improve the performance and reliability of long-running scripts, particularly in a multi-user environment.
The Script
Below is a sample Bash script that utilizes the flock command to manage file locks. This script is designed to prevent overlapping executions by locking a designated file:
#!/bin/bash
LOCKFILE="/tmp/myscript.lock"
exec 200>$LOCKFILE # Open the lock file with file descriptor 200
flock -n 200 || {
echo "Another instance is already running."
exit 1
}
# Place the main script logic here
echo "Script is running..."
sleep 20 # Simulate a long-running process
echo "Script has completed."
# The lock will be automatically released when the script exits
Step-by-Step Explanation
NOTE!
Ensure the lock file’s path is writable and that you have permission to execute the script.
In this section, we will break down the script into its fundamental parts, detailing each step of the process.
- Define the Lock File: Use a temporary file to serve as a lock (e.g.,
/tmp/myscript.lock
). This is where the flock command will check for existing locks. - Use flock with a File Descriptor: The script will execute within a block that opens the lock file as a file descriptor (200 in this case). This ensures that the enclosed commands are executed in a locked context.
- Handle Concurrent Executions: If another instance of the script tries to run while the lock is active, it will print a message and exit gracefully.
- Implement Main Logic: Inside the locked block, insert the primary operations that the script should perform, ensuring that these actions cannot be disturbed by concurrent executions.
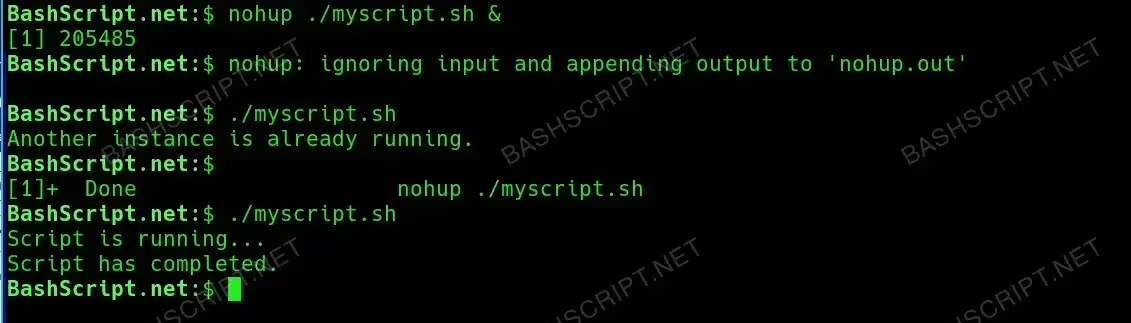
How to Run the Script
To execute the Bash script effectively, follow these steps:
- Open your terminal.
- Copy the script code and save it in a file (e.g.,
myscript.sh
). - Make the script executable by running:
chmod +x myscript.sh
- Run the script with:
./myscript.sh
Conclusion
Using flock in your Bash scripts is an invaluable technique for managing locks and preventing overlapping processes. With this method, you can ensure that your scripts run smoothly without the risk of contention and redundancy. By implementing file locks, you enhance the overall stability and reliability of your automated tasks.
FAQ
-
What happens if my script exits unexpectedly?
If your script exits unexpectedly, the lock file will remain even after the process ends. Consider adding cleanup logic to remove the lock file under specific conditions.
-
Can multiple scripts share the same lock file?
Yes, multiple scripts can use the same lock file to coordinate their executions. Just ensure that they correctly manage what they are protecting.
-
Is flock available on all Unix-like systems?
Most modern Unix-like systems include flock by default, but you may need to install it on some versions manually.
-
How can I test if my locking is working?
You can test this by running the script in multiple terminal sessions simultaneously. You should see the warning message indicating that another instance is running.
Troubleshooting
If you encounter issues while using flock, here are some common problems and their fixes:
- Issue: “Another instance is already running” message appears.
Fix: Ensure that no other instances of the script are active, or wait for the current execution to finish. - Issue: Lock file is not removed when the script exits.
Fix: Add proper error handling to remove the lock file upon exit or consider using a trap to handle signals.