When it comes to automating tasks in a Linux environment, the Bash scripting language is an incredibly powerful tool. One of the fundamental structures you’ll encounter in Bash is the while loop. This article will explore a basic example of a while loop in a Bash script, helping you understand how it functions and how you can use it in your own scripts.
Prerequisites
- You should have a basic understanding of Bash syntax.
- Familiarity with variables and conditions in Bash.
- Understanding of looping constructs.
- Access to a terminal with the Bash shell installed.
DID YOU KNOW?
The while loop will continue to execute as long as its designated condition evaluates to true. This makes it essential for repetitive tasks!
The Script
This simple script demonstrates how a while loop can be used to count from 1 to 5. The script initializes a variable, checks a condition, and increments the variable until it reaches the specified limit.
#!/bin/bash
count=1
while [ $count -le 5 ]
do
echo "Count: $count"
((count++))
done
Step-by-Step Explanation
NOTE!
This script is a simple demonstration, but while loops can be used for more complex tasks such as processing files, waiting for conditions, or running background tasks depending on conditions.
Let’s break down the script into its core components:
- Variable Initialization: The script starts by defining a variable
count
and initializes it to 1. - Defining the Condition: The
while
statement checks ifcount
is less than or equal to 5. - Executing the Loop: Inside the do-done block, the script echoes the current count.
- Incrementing the Count: The
count
variable is incremented by 1 in each iteration.
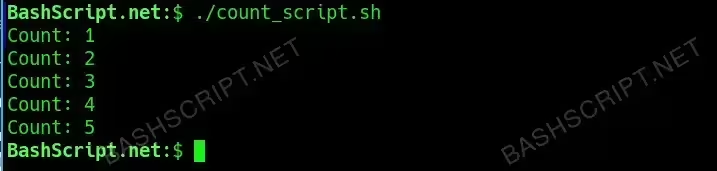
How to Run the Script
To execute this Bash script, follow these simple steps:
- Create a new file using
nano count_script.sh
. - Copy and paste the above script into the file and save it.
- Run the script using the command
bash count_script.sh
in your terminal.
Conclusion
In this article, we explored a basic while loop in a Bash script, demonstrating how to use it to perform repetitive actions. Mastering loops is crucial for writing efficient scripts that can automate mundane tasks in your daily workflow, enhancing your productivity in a Linux environment.
FAQ
-
What is a while loop?
A while loop is a control flow statement that allows code to be executed repeatedly based on a given condition.
-
Can I use other conditions in a while loop?
Yes, you can use any valid comparisons or commands that return an exit status in the while loop condition.
-
What happens if the condition is always true?
If the condition is always true, the loop will run indefinitely, leading to an infinite loop. Ensure your conditions are set correctly to avoid this.
-
How can I exit a while loop early?
You can use the
break
command within the loop whenever you want to exit before the condition is false.
Troubleshooting
Here are some common errors you may encounter when running a while loop in Bash scripts, along with their solutions:
- Syntax error: Ensure that your syntax is correct, and that you’ve paired braces and brackets properly.
- Infinite loops: Use the
break
command effectively to exit if necessary, or verify that your loop’s condition will eventually evaluate to false. - Permission denied: Make sure your script file has permission to be executed. Run
chmod +x count_script.sh
to make it executable.