Writing Bash scripts can significantly enhance your productivity, especially when performing repetitive tasks. This article provides a step-by-step guide on creating a Bash script that features a built-in help menu and usage examples, making it user-friendly for both beginners and advanced users. By incorporating essential elements such as a help function, comments for clarity, and command-line argument parsing, you will create a script that is not only functional but also easy to understand and maintain.
Prerequisites
- Basic understanding of the Bash shell and command-line operations.
- Familiarity with variables, conditional statements, loops, and functions in Bash.
- Knowledge of how to create and edit text files using a text editor.
- No additional packages are required; however, having a Bash-compatible terminal is recommended.
DID YOU KNOW?
Shell scripts are powerful tools for automating system tasks and can save you a lot of time when dealing with repetitive processes!
The Script
The following script serves as a foundation for creating user-friendly Bash scripts. It includes a help function that displays usage instructions, making it accessible to users who invoke it without arguments or with the `–help` flag.
#!/bin/bash
# A simple script with help menu
function show_help() {
echo "Usage: script.sh [OPTIONS]"
echo "Options:"
echo " --help Display this help message"
echo " --version Show the script version"
}
# Check if no arguments are provided
if [ "$#" -eq 0 ]; then
show_help
exit 1
fi
while [[ "$1" != "" ]]; do
case $1 in
--help )
show_help
exit
;;
--version )
echo "Script Version 1.0"
exit
;;
* )
echo "Invalid option: $1"
show_help
exit 1
esac
shift
done
Step-by-Step Explanation
NOTE!
Each section of the script serves a specific purpose, and understanding these is crucial for modifying or extending its functionality.
Let’s break down the script into manageable parts:
- Shebang Line: The line
#!/bin/bash
at the top of the script informs the system that this file should be executed using the Bash shell. - Help Function: The
show_help
function prints out usage instructions. This is critical for user-friendliness and assists in guiding users through the script’s options. - Argument Check: The script checks if any arguments are passed. If not, it calls the help function and exits.
- Option Parsing: A while loop processes each command-line argument using a case statement. This allows the script to handle various options, providing feedback based on user input.
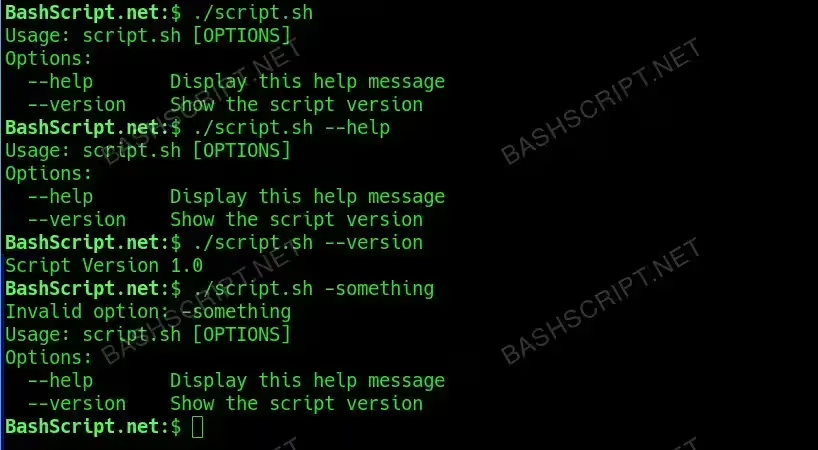
How to Run the Script
To execute your newly created Bash script, follow these steps:
- Open your terminal.
- Navigate to the directory where your script is located using the
cd
command. - Make the script executable with the command:
chmod +x script.sh
. - Run the script using:
./script.sh
. You can also provide options like./script.sh --help
or./script.sh --version
.
Conclusion
By following this guide, you now have a reusable template for writing professional-grade Bash scripts that include clear documentation and robust functionality. With built-in help options, your scripts will be accessible to users of all skill levels.
FAQ
-
What if I forget to provide an argument?
The script will display the help message, guiding you on how to use it properly.
-
Can I add more options to the script?
Absolutely! You can modify the
case
statement in the argument parsing section to add more options as needed. -
How do I check if my script works?
After running the script with various options, check that it responds correctly to the inputs you provide.
-
What is the purpose of comments in the script?
Comments serve to explain sections of the script, making it easier for others (and yourself) to understand the code later.
Troubleshooting
Here are some common error messages you might encounter and how to resolve them:
- permission denied: Ensure that you have made the script executable with
chmod +x script.sh
. - command not found: Verify that you are in the correct directory and that the script filename is correct.
- invalid option: This indicates that the script received an unrecognized argument. Double-check your inputs against the available options.