Setting colors in your Bash scripts can significantly enhance the visual output, making it easier for users to follow along. In this article, we will explore how to set different colors in your Bash scripts to highlight information, errors, or simply to beautify the console output.
Prerequisites
DID YOU KNOW?
The escape sequences used for colors in the terminal are ANSI escape codes. These codes allow for a rich set of formatting options!
The Script
Below is an example script that demonstrates how to set colors for different outputs in the terminal. You can use these color codes to format your terminal output.
#!/bin/bash
# Define color codes
RED='\033[0;31m'
GREEN='\033[0;32m'
YELLOW='\033[1;33m'
BLUE='\033[0;34m'
NC='\033[0m' # No Color
# Use colors in your outputs
echo -e "${GREEN}Success: Everything loaded correctly!${NC}"
echo -e "${YELLOW}Warning: This is a warning message.${NC}"
echo -e "${RED}Error: Something went wrong!${NC}"
Step-by-Step Explanation
NOTE!
When using color codes, remember to reset the color back to normal using the ${NC}
code to avoid affecting other terminal outputs.
Here’s a breakdown of the script components:
- Color Definitions: The first step is to define your color codes. Each color is defined using ANSI escape sequences, such as
'\033[0;31m'
for red. - Output with Color: To use these colors, you simply include the color code in your
echo
statements. The-e
flag allows echo to interpret backslash escapes. - Resetting Color: After each color output, it’s important to reset the color to normal by appending
${NC}
to your outputs.
How to Run the Script
To execute your Bash script, follow these steps:
- Create a new file using your favorite text editor, for example,
nano color_script.sh
. - Copy and paste the script into your file and save it.
- Make the script executable by running
chmod +x color_script.sh
in the terminal. - Run the script with
./color_script.sh
.
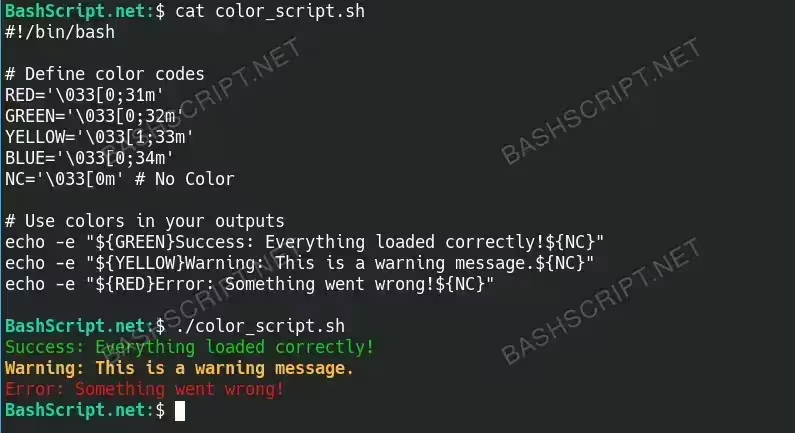
Conclusion
By using colors in your Bash script output, you can create a more engaging user experience and make it much easier to process output information quickly. Experiment with different colors and styles to find what works best for your scripts!
FAQ
-
Can I use colors in scripts run on all terminals?
Most modern terminals support ANSI colors, but some older ones may not. Test in your specific terminal.
-
How do I know what ANSI codes correspond to which colors?
You can find a chart online that maps ANSI codes to colors or check the documentation for your terminal.
-
Can I change the style as well as the color?
Yes! You can also use codes for bold, underline, and other text styles along with color codes.
-
What happens if I forget to reset the color?
If you forget to reset the color using
${NC}
, all subsequent text in the terminal will continue to be colored until reset, which might confuse users.
Troubleshooting
Here are some common errors related to color codes in Bash scripts and how to resolve them:
- Unrecognized escape sequence: Ensure that you are using the
-e
flag withecho
. For example,echo -e "${RED}Text"
. - Color not changing: Check if your terminal supports ANSI colors or if you are outputting text to a non-terminal interface.
- Script not executing: Make sure you have set execute permissions using
chmod +x script.sh
.