In today’s computing world, understanding the specifications of your hardware can be crucial for optimizing performance, especially when it comes to multiprocessing tasks. One important aspect is knowing how many CPU cores and sockets your system has. This can help you assess how well your system can handle parallel operations. In this article, we will discuss a simple Bash script that you can use to count the CPU cores and sockets on your Linux machine.
Prerequisites
- Basic knowledge of Bash scripting
- Understanding of variables and functions in Bash
- Familiarity with system commands such as
lscpu
- Privilege to execute scripts on your Linux system
DID YOU KNOW?
The number of CPU cores directly impacts the performance of parallel processes, making it essential for applications that require high computing power.
The Script
This script will retrieve information about the CPU using the lscpu
command, which is commonly available on Linux systems. It extracts the number of sockets and cores available on your CPU.
#!/bin/bash
# Get the number of sockets
sockets=$(lscpu | grep 'Socket(s):' | awk '{print $2}')
# Get the number of cores
cores=$(lscpu | grep 'Core(s) per socket:' | awk '{print $4}')
echo "Number of sockets: $sockets"
echo "Number of cores per socket: $cores"
echo "Total number of cores: $(($sockets * $cores))"
Step-by-Step Explanation
NOTE!
Ensure that the lscpu
command is installed and accessible on your Linux distribution.
In this section, we will break down the script to understand how it works:
- Define the Script: The script begins with a shebang (#!/bin/bash), indicating that it should be run using Bash.
- Retrieve Sockets: It uses the command
lscpu
to get the number of sockets available in the system by searching for ‘Socket(s):’. - Retrieve Cores: Similarly, it extracts the number of cores per socket using ‘Core(s) per socket:’.
- Display Results: Finally, it prints the total number of sockets, cores per socket, and calculates the total number of cores by multiplying the two values.
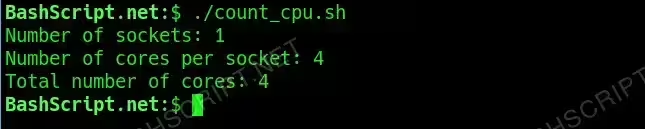
How to Run the Script
Running the script is straightforward. Follow these steps:
- Create a new file and paste the script into it. For example, you could name it
count_cpu.sh
. - Make the script executable by running
chmod +x count_cpu.sh
. - Execute the script using
./count_cpu.sh
in your terminal.
Conclusion
Knowing the number of CPU cores and sockets on your system can greatly aid in making decisions on performance optimization for various tasks. With the provided Bash script, you can quickly gather this information without delving into complex configurations. Modify the script as needed for more detailed outputs based on your requirements.
FAQ
-
What if the script fails to execute?
Ensure that you have the correct permissions and that the
lscpu
command is installed on your system. -
Can this script run on all Linux distributions?
Yes, as long as the
lscpu
command is available, the script should work on any Linux distribution. -
How can I modify the script to include more details?
You can customize the
lscpu
command to retrieve additional information by modifying the grep statements in the script. -
Why is it important to know the number of cores?
Knowing the number of cores helps to optimize tasks that can be parallelized and improves overall system performance.
Troubleshooting
Here are some common errors you might encounter and how to fix them:
- Command not found: Ensure that the
lscpu
command is installed. You can install it using your package manager. - Permission denied: Make sure you have made the script executable using
chmod +x
. - No output: Check your script for any syntax errors and make sure the
lscpu
command is returning results.