Creating a simple countdown timer can be a fun and practical project for anyone learning Bash scripting. In this article, we will guide you through the process of creating your very own countdown timer using a Bash script. This script can be used for various tasks, such as timing your cooking, workouts, or any activities that require precise tracking.
Prerequisites
- Bash basics: Familiarity with variables, control structures, and functions.
- Understanding of loops: You’ll need to utilize loops in your script.
- Terminal experience: Comfort with executing commands in a terminal environment.
DID YOU KNOW?
Bash scripting is widely used in the Linux environment for automating tasks and managing system processes.
The Script
The countdown timer script is straightforward. It prompts you for the number of seconds and then counts down to zero, displaying each second. Below is the complete Bash script:
#!/bin/bash
read -p "Enter time in seconds: " seconds
while [ $seconds -gt 0 ]; do
echo "$seconds"
sleep 1
seconds=$((seconds - 1))
done
echo "Time's up!"
Step-by-Step Explanation
NOTE!
Make sure to give the script execute permissions before running it with the command: chmod +x script_name.sh
Now let’s break down the script to understand how it works:
- Reading input: We use
read
to take the number of seconds from the user. - Looping through countdown: A
while
loop continues until seconds reach zero. - Displaying countdown: Each second is printed to the terminal, then the script sleeps for 1 second before reducing the countdown.
- Completion message: Once the countdown finishes, a message “Time’s up!” is displayed.
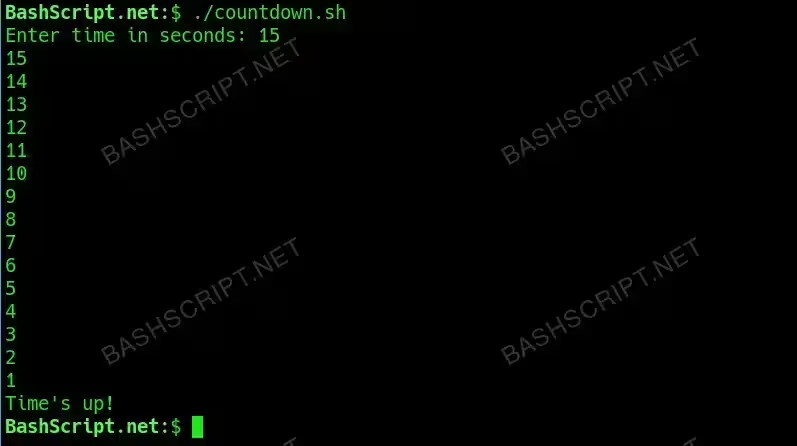
How to Run the Script
To run the countdown timer script, follow these simple steps:
- Open your terminal.
- Navigate to the directory where your script is saved.
- Execute the script by running:
./script_name.sh
and follow the prompt to enter the time in seconds.
Conclusion
Creating a countdown timer in Bash is a simple yet effective way to practice scripting. This exercise reinforces your understanding of loops, variables, and handling user input in Bash. With the skills learned here, you can expand upon this script, perhaps adding features like alarms or logging the countdown to a file.
FAQ
-
Can I use this script on Windows?
Yes, but you need to have Bash installed, such as through WSL (Windows Subsystem for Linux) or other environments like Git Bash.
-
What happens if I enter a non-numeric value?
The script will likely return an error or behave unexpectedly. Input validation can be added to handle such cases.
-
Can I change the countdown interval?
Yes! You can modify the
sleep 1
command to a different number of seconds for longer intervals. -
How do I stop the countdown once it has started?
You can usually interrupt the script by pressing
Ctrl + C
in the terminal. -
Can I customize the start message?
Absolutely! You can replace the
echo
statement in the script to output any message you’d like.
Troubleshooting
- Error: permission denied: Make sure to give the script execute permissions using
chmod +x script_name.sh
. - Error: command not found: Ensure that you’re in the correct directory and that the script file exists.
- Unexpected results: Ensure that the input is numeric; consider adding input validation to the script.