Bash scripting is a powerful way to automate tasks and improve productivity in a UNIX-like environment. In this article, we will explore how to create a minimal Bash script that accepts optional input arguments. By the end, you will understand how to leverage input to enhance the functionality of your scripts.
Prerequisites
- Basic understanding of Bash commands.
- Knowledge of variables in Bash.
- Experience with functions and conditional statements.
- Familiarity with running scripts in a terminal environment.
DID YOU KNOW?
Bash stands for “Bourne Again SHell,” a play on words referencing the original Bourne shell, which was created by Stephen Bourne.
The Script
Below is a simple Bash script example that demonstrates how to use optional input arguments. If no arguments are provided, it defaults to a predefined value.
#!/bin/bash
# Get the first argument or default to "World"
name="${1:-World}"
echo "Hello, $name!"
Step-by-Step Explanation
NOTE!
Make sure to give execution permission to your script using chmod +x script.sh
before running it.
Let’s break down the script to understand its components:
- Shebang Line: The first line
#!/bin/bash
defines the script’s interpreter. - Capture Input: The line
name="${1:-World}"
captures the first input argument or defaults to “World” if not provided. - Echo Command: Finally,
echo "Hello, $name!"
outputs the greeting based on user input.
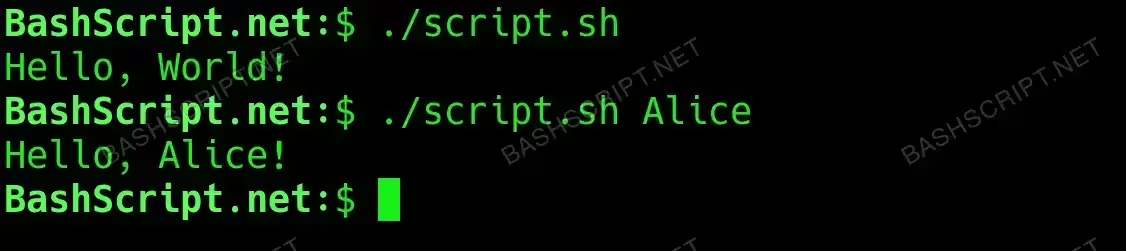
How to Run the Script
To execute the script, follow these simple steps:
- Open your terminal.
- Navigate to the directory containing the script using
cd
. - Run the script with optional arguments, for example:
./script.sh Alice
. If no argument is given, it will greet “World”.
Conclusion
By utilizing optional input arguments in your Bash scripts, you can create more versatile and user-friendly scripts. This example serves as a foundation for further exploration and enhancement of your scripting skills.
FAQ
-
What happens if I run the script without any arguments?
The script will greet “World” as the default name.
-
Can I customize the default name in the script?
Yes, you can change “World” to any other string you wish.
-
How do I provide multiple arguments?
You can expand the script to handle multiple arguments, but this basic example only utilizes the first argument.
-
Is this script portable across different UNIX/Linux systems?
Yes, as long as Bash is installed, this script will work across various UNIX/Linux distributions.
-
What if I receive an error when running the script?
Make sure the script has execution permission set, and you are in the correct directory.
Troubleshooting
Here are some common error messages you might encounter and how to resolve them:
- Permission denied: This means the script does not have execute permissions. Use
chmod +x script.sh
to fix it. - command not found: Ensure you’re using the correct path to your script, or that the script is where you think it is.
- unexpected token: This can happen if there are syntax errors in your script. Double-check the code for mistakes.