In today’s digital world, managing files efficiently is crucial. One common task is zipping files within a directory to save space or to prepare for sharing. This article will guide you through creating a Bash script that zips all files in a specified directory. The script will accept the directory as a command-line argument, extract the directory name, and generate a zip file named with both the original directory name and the current date and time. Let’s get started!
Prerequisites
- Basic understanding of Bash scripting
- Familiarity with the command line interface
- Knowledge of
zip
command and its usage - Ensure zip package is installed (you can install it using
sudo apt-get install zip
on Debian-based systems)
DID YOU KNOW?
The zip
utility was created in 1989 and is now one of the most popular compression formats used today!
The Script
This script will use a simple logic to create a zip file from all the contents of a specified directory. Here’s how it works:
#!/bin/bash
dir="$1"
timestamp=$(date "+%Y%m%d_%H%M%S")
dirname=$(basename "$dir")
zipfile="${dirname}_${timestamp}.zip"
zip -r "$zipfile" "$dir"
Step-by-Step Explanation
NOTE!
Before running the script, make sure you give it the appropriate permissions by executing chmod +x zip_files.sh
.
Let’s break down the script into understandable parts:
- Extracting the Directory: The argument provided is stored in the variable
dir
. This represents the directory that you want to zip. - Getting Current Timestamp: The
date
command is utilized to get the current date and time in the formatYYYYMMDD_HHMMSS
. This is stored in thetimestamp
variable. - Extracting Base Directory Name: The
basename
command is used to get the last part of the directory path (the actual directory name) and is stored in thedirname
variable. - Creating the Zip File: The
zip
command compresses the specified directory recursively (-r
) and names the output file using the extracted directory name and the current timestamp.
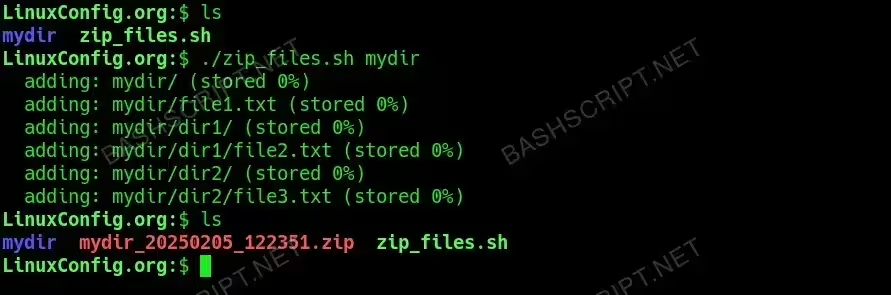
How to Run the Script
Once the script is saved and permissions are set, follow these steps to execute it:
- Open your terminal.
- Navigate to the directory where your Bash script is located.
- Run the script with the target directory as an argument, like so:
./zip_files.sh /path/to/directory
.
Conclusion
This Bash script provides a simple yet effective way to zip all files in a directory while automatically naming the output file with the current date and time. It’s perfect for backing up files or preparing them for transfer. By following this guide, you can easily customize and extend the script to suit your specific needs.
FAQ
-
What if the directory does not exist?
The script will fail and indicate that the directory is not found. Always ensure the path is correct.
-
Can I zip only specific file types?
Yes! You can modify the
zip
command to include filters for specific file types if necessary. -
Is there a limit on the number of files I can zip?
The limit usually depends on your system resources, but zip can handle a large number of files quite efficiently.
-
Can I run this script on a different operating system?
This script is intended for Unix-like systems. If you’re on Windows, consider using WSL (Windows Subsystem for Linux) or similar tools.
Troubleshooting
Here are some common errors you might encounter and how to resolve them:
- Error: Permission Denied – Make sure you give your script execute permissions with
chmod +x script_name.sh
. - Error: Directory Not Found – Double-check the path you provided to the script. Ensure there’s no typo.
- Error: zip command not found – Install the zip package using your package manager (e.g.,
sudo apt-get install zip
).