In today’s data-driven world, converting data between formats is a common task. One such conversion that many developers face is changing JSON data into the more spreadsheet-friendly CSV format. This article provides a straightforward Bash script to perform this conversion efficiently. We will also explore a sample JSON data structure for clarity.
Prerequisites
- Bash scripting knowledge – Familiarity with variables, loops, and conditionals.
- jq – A lightweight and flexible command-line JSON processor.
- Basic command-line skills – Understanding how to execute scripts and interpret output.
DID YOU KNOW?
JSON stands for JavaScript Object Notation and is widely used for data interchange on the web due to its lightweight nature.
The Script
This Bash script leverages jq
to parse the JSON input and convert it into CSV format. The following script assumes a simple JSON structure for demonstration purposes.
#!/bin/bash
# Check if the input file is provided
if [ "$#" -ne 2 ]; then
echo "Usage: $0 input.json output.csv"
exit 1
fi
# Convert JSON to CSV
jq -r '(.[] | [."name", ."age", ."city"] | @csv)' "$1" > "$2"
Step-by-Step Explanation
NOTE!
This script expects a specific JSON format. Make sure your JSON follows a similar structure as shown in the example below.
Here’s how the script works:
- Input Validation: The script first checks if the user has provided the required input and output file names. If not, it displays usage instructions.
- JSON Parsing: Using
jq
, the script parses the input JSON. It selects the fields to be converted. - CSV Conversion: The selected fields from each JSON object are formatted as CSV and saved to the specified output file.
The script is designed to handle a JSON array of objects. Here’s an example of what the input JSON might look like:
[
{"name": "John Doe", "age": 30, "city": "New York"},
{"name": "Jane Smith", "age": 25, "city": "Los Angeles"},
{"name": "Sam Green", "age": 35, "city": "Chicago"}
]
How to Run the Script
To run the script, follow these steps:
- Open your terminal. Navigate to the directory where your script and JSON file are located.
- Make the script executable: Run
chmod +x your_script.sh
to give execute permissions. - Execute the script: Use the command
./your_script.sh input.json output.csv
to convert your JSON data.
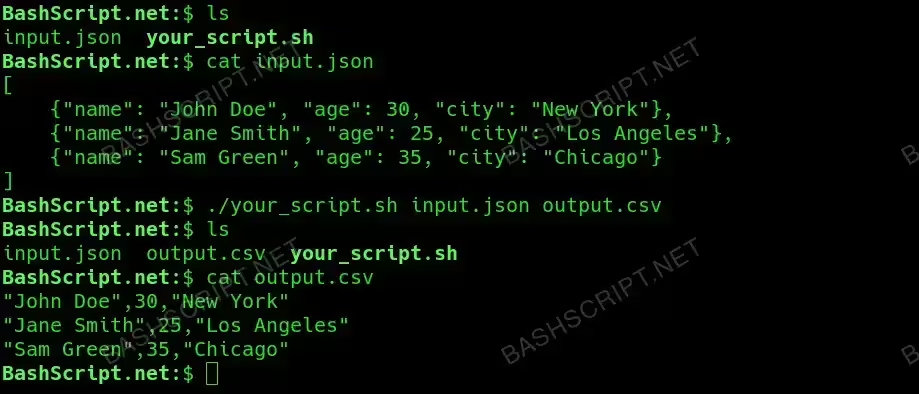
Conclusion
Converting JSON to CSV can be accomplished easily with a simple Bash script and the jq
tool. By following the steps outlined in this article, you can automate the conversion process and leverage your data efficiently.
FAQ
-
What if my JSON has a different structure?
You will need to adjust the
jq
command in the script to match the structure of your JSON data. -
Can I convert nested JSON objects?
Yes, but the
jq
command will need to be more complex to navigate the nested structure. -
Is there any JSON structure limitation with this script?
The script assumes that each item in the JSON array has the same keys. Variations in keys may need additional handling.
-
What happens if the output file already exists?
The script will overwrite the existing output file without warning.
-
Where can I learn more about
jq
?You can visit the jq website for comprehensive documentation and examples.
Troubleshooting
Here are some common errors that you might encounter and how to resolve them:
- Error: “jq: command not found” – This indicates that
jq
is not installed. Install it using your package manager, e.g.,sudo apt-get install jq
on Ubuntu. - Error: “Usage: ./script.sh input.json output.csv” – This means you did not provide the correct number of arguments. Ensure you are passing both input and output file names.
- Error: “input.json: No such file or directory” – Verify that the JSON file path is correct and that the file exists.