In the ever-evolving world of cryptocurrency, staying updated with the latest prices is crucial for traders and enthusiasts alike. This article will guide you through creating a simple Bash script that checks the price of Bitcoin (BTC) in USD and provides information on additional cryptocurrencies like Ethereum (ETH), Zcash (ZEC), and Litecoin (LTC) using a free API.
Prerequisites
- Basic understanding of Bash scripting
- Familiarity with API requests and JSON formatting
- Have curl installed on your system
- Ensure you have a working internet connection
DID YOU KNOW?
Bitcoin was the first decentralized cryptocurrency, created in 2009 by an unknown person or group of people using the pseudonym Satoshi Nakamoto.
The Script
This script will fetch real-time cryptocurrency prices from the CoinGecko API
. It uses curl
to send an HTTP request and parse the JSON response to display the current Bitcoin price along with the prices of Ethereum, Zcash, and Litecoin.
#!/bin/bash
# Fetching cryptocurrency prices
response=$(curl -s 'https://api.coingecko.com/api/v3/simple/price?ids=bitcoin,ethereum,zcash,litecoin&vs_currencies=usd')
# Extracting prices
btc_price=$(echo $response | jq .bitcoin.usd)
eth_price=$(echo $response | jq .ethereum.usd)
zec_price=$(echo $response | jq .zcash.usd)
ltc_price=$(echo $response | jq .litecoin.usd)
# Displaying prices
echo "Current Bitcoin Price: $btc_price USD"
echo "Current Ethereum Price: $eth_price USD"
echo "Current Zcash Price: $zec_price USD"
echo "Current Litecoin Price: $ltc_price USD"
Step-by-Step Explanation
NOTE!
Make sure to have jq
installed to parse JSON responses. You can install it using your package manager.
Let’s break down the script step by step:
- Fetch Prices: We make a request to the
CoinGecko API
to fetch the current prices of Bitcoin and other cryptocurrencies. - Parse JSON: The response is a JSON object, which we parse using
jq
to extract the relevant prices. - Display Results: Finally, we print the prices to the terminal.
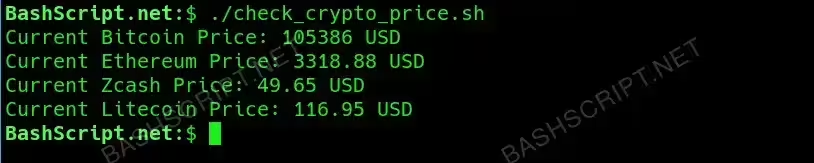
How to Run the Script
To successfully execute the script, follow these steps:
- Open a terminal window on your system.
- Create a new Bash script file using
nano check_crypto_price.sh
. - Copy and paste the above script into the file, then save and exit.
- Make the script executable with
chmod +x check_crypto_price.sh
. - Run the script using
./check_crypto_price.sh
.
Conclusion
This simple Bash script enables you to quickly check the price of Bitcoin and a selection of other cryptocurrencies in USD. By utilizing the CoinGecko API
, it provides a handy way to stay informed about cryptocurrency market trends.
FAQ
-
What if I don’t have
curl
installed?You can install
curl
using your package manager. For example, on Ubuntu, you can runsudo apt install curl
. -
Can I add more cryptocurrencies?
Yes! Just append their IDs to the API URL, separated by commas. Refer to CoinGecko’s API documentation for available IDs.
-
What is
jq
and do I need it?jq
is a lightweight command-line JSON processor. It is needed to parse the API response. You can install it via your package manager. -
Is there a limit to API requests?
Yes, APIs often have rate limits. Check the CoinGecko documentation for details on their limits.
-
What if I get an error message when running the script?
Ensure you have
curl
andjq
installed correctly. Check your internet connection as well.
Troubleshooting
If you encounter any issues while running the script, consider the following common problems:
- Command not found: Make sure
curl
andjq
are installed. - No internet connection: Verify your network settings.
- API request fails: The API might be down or unavailable. Try again later.