case
statements to replicate the behavior of jumping between labels, offering a cleaner and more maintainable alternative to traditional goto logic.
Prerequisites
- Basic understanding of Bash scripting
- Knowledge of
case
statements - Familiarity with loops (e.g.,
while
) - A Linux or macOS environment with Bash installed
DID YOU KNOW?
Bash doesn’t natively support goto because it promotes structured programming, which is easier to read and debug.
The Script
This script simulates a goto-like behavior using a variable to track the current “label” and a case
statement to jump between sections.
#!/bin/bash
label="start"
while true; do
case $label in
start)
echo "This is the start label."
echo "Where do you want to go? (next/end)"
read -r choice
if [[ $choice == "next" ]]; then
label="next"
elif [[ $choice == "end" ]]; then
label="end"
else
echo "Invalid choice. Staying at start."
fi
;;
next)
echo "You are now at the next label."
echo "Where to now? (start/end)"
read -r choice
if [[ $choice == "start" ]]; then
label="start"
elif [[ $choice == "end" ]]; then
label="end"
else
echo "Invalid choice. Staying at next."
fi
;;
end)
echo "You have reached the end. Exiting..."
break
;;
*)
echo "Unknown label. Exiting..."
break
;;
esac
done
Step-by-Step Explanation
NOTE!
This approach avoids the pitfalls of traditional goto statements, keeping the code more readable and maintainable.
Here’s how the script works, broken down step-by-step:
- Initialize the Label: Set the initial label to “start” using a variable.
- Create a Loop: Use a
while true
loop to allow repeated execution of thecase
statement. - Define Case Labels: Create branches in the
case
statement for each label (e.g.,start
,next
,end
). - Handle User Input: Use
read
to capture user input and update the label variable accordingly. - Exit Gracefully: Break the loop when the label is “end” or invalid.
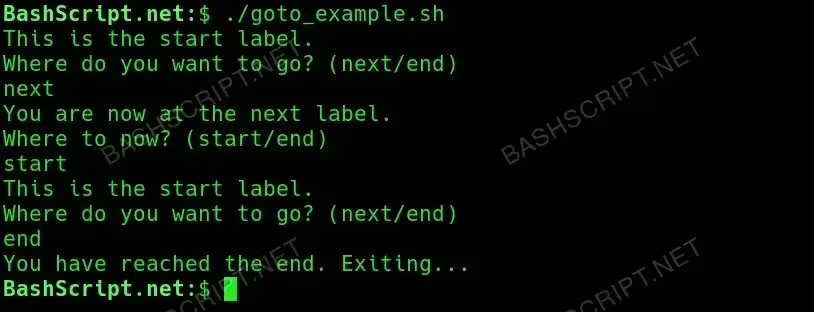
How to Run the Script
Follow these steps to execute the script:
- Save the script to a file, e.g.,
goto_example.sh
. - Make the script executable by running
chmod +x goto_example.sh
. - Run the script using
./goto_example.sh
.
Conclusion
Simulating goto in Bash using structured techniques like case
statements provides a cleaner and more maintainable way to control script flow. This approach is versatile and avoids the common pitfalls of traditional goto logic.
FAQ
-
Why doesn’t Bash support goto natively?
Bash promotes structured programming, which is easier to read, debug, and maintain compared to unstructured goto logic.
-
Can I use this technique in other shells?
Yes, this approach can be adapted for other shells like Zsh, as long as they support
case
statements and loops. -
What happens if an invalid label is entered?
The script will display an error message and exit gracefully.
-
Can this approach handle complex scripts?
Yes, this technique is scalable and can handle complex flow control with multiple labels.
Troubleshooting
- Error:
Permission denied
Fix: Ensure the script is executable by runningchmod +x filename.sh
. - Error:
Command not found
Fix: Verify that Bash is installed and the script is being executed with the correct path.