In the world of network management, switching between different name servers can be a common task. Whether you are troubleshooting DNS issues or simply switching providers, having an automated way to toggle between two name servers can save time and effort. This article will guide you through creating a simple Bash script that allows you to easily switch between two hard-coded name servers in the /etc/resolv.conf
file.
Prerequisites
- Bash programming fundamentals: Understanding variables, functions, and control statements.
- Basic knowledge of
resolv.conf
file and its structure. - Access to a terminal with appropriate permissions to modify system files.
- Root or sudo privileges to edit the
/etc/resolv.conf
file.
DID YOU KNOW?
Changing name servers can significantly improve your Internet speed and security depending on the provider you choose.
The Script
The following script defines two name server IP addresses as constants and provides a mechanism to switch between them. This script reads the current name server from /etc/resolv.conf
and switches to the other one whenever executed.
#!/bin/bash
# Constants for name servers
NS1="8.8.8.8"
NS2="1.1.1.1"
# Get the current name server
CURRENT_NS=$(grep -Eo '^[^#]*' /etc/resolv.conf | awk '/^nameserver /{print $2}')
# Switch name servers
if [ "$CURRENT_NS" == "$NS1" ]; then
sed -i "s/$CURRENT_NS/$NS2/" /etc/resolv.conf
echo "Switched to Name Server: $NS2"
else
sed -i "s/$CURRENT_NS/$NS1/" /etc/resolv.conf
echo "Switched to Name Server: $NS1"
fi
Step-by-Step Explanation
NOTE!
This script manipulates the critical /etc/resolv.conf
file. Ensure you have a backup before running it.
Now let’s break down how this script functions.
- Define Constants: The script starts by defining two constants,
NS1
andNS2
, which represent the IP addresses of the name servers you want to switch between. - Get Current Name Server: The script retrieves the currently active name server by reading
/etc/resolv.conf
usinggrep
andawk
. - Switch Logic: The script compares the current name server to determine which server to switch to. It uses a simple
<a href="https://bashscript.net/bash-script-conditional-checks/">if</a>
statement to check this condition and updates the file accordingly. - Output: The script prints a message to the terminal indicating which name server is currently active after the change.
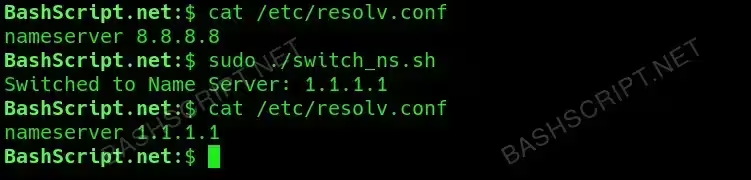
How to Run the Script
To run the script successfully, follow these steps:
- Open your terminal.
- Navigate to the directory where the script is saved.
- Run the script using the command
sudo bash switch_ns.sh
, replacingswitch_ns.sh
with your script’s filename.
Conclusion
Switching between name servers can be an important technique for network management, often aiding in resolving connectivity issues. This simple Bash script automates the process, ensuring you can rapidly switch without manually editing configuration files. Remember to always backup your files before running scripts that modify system configurations.
FAQ
-
Can I modify the script for more than two name servers?
Yes, you can extend the script with additional server variables and modify the switching logic accordingly.
-
What happens if I do not have sudo privileges?
Without sudo privileges, you will not be able to modify
/etc/resolv.conf
. You will need to run the script as a user with the necessary permissions. -
What if the script doesn’t work as intended?
Check for syntax errors and ensure you are running the script with appropriate permissions. Reviewing terminal output messages can also provide clues.
-
How can I find out what my current DNS server is?
You can check your current DNS settings by looking at the file
/etc/resolv.conf
using the commandcat /etc/resolv.conf
.
Troubleshooting
Here are some common issues you might encounter and how to resolve them:
- Error: Permission denied – Ensure you are running the script with
sudo
to have the necessary permissions. - Incorrect server information – Double-check the IP addresses defined in the script constants to make sure they are valid and correctly formatted.
- Script not executing – Make sure the script has execute permissions. You can set this with the command
chmod +x switch_ns.sh
.