In the world of programming, manipulating strings is a common task. Whether you’re processing data or automating tasks, being able to remove the last character from a string can be quite useful. This article will guide you through creating a simple Bash script to achieve this.
Prerequisites
- Basic knowledge of Bash scripting
- Understanding of variables and string manipulation
- Familiarity with using the command line interface
- No additional packages are required; Bash is sufficient
DID YOU KNOW?
In Bash, strings are often a series of characters enclosed in quotes. Removing the last character is a simple yet common requirement in many scripts!
The Script
This script defines a variable to hold your string and then employs parameter expansion to remove the last character. The following is a simple script to illustrate this:
#!/bin/bash
string="Hello, World!"
echo "Original String: $string"
modified_string=${string::-1}
echo "Modified String: $modified_string"
Step-by-Step Explanation
NOTE!
Make sure to give your script execution permissions using chmod +x script.sh
before running it.
Here’s a breakdown of how this script operates:
- Define the String: The string “Hello, World!” is assigned to the variable
string
. - Display the Original String: The script echoes the original string to the console.
- Remove Last Character: Using parameter expansion with
${string::-1}
, the last character is removed from the string. - Display the Modified String: Finally, the modified string is displayed on the console.
How to Run the Script
To execute the script, follow these steps:
- Open your terminal application.
- Navigate to the directory where your script is saved using
cd path/to/directory
. - Run the script with
./script.sh
.
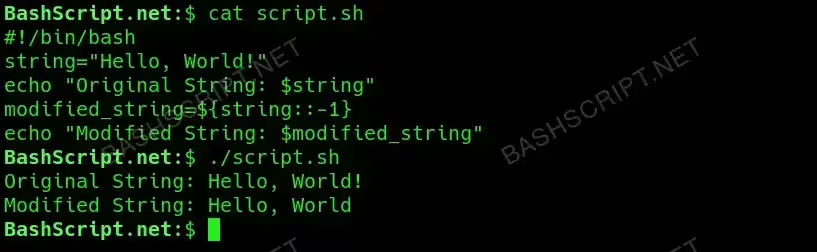
Conclusion
Removing the last character from a string in Bash is straightforward with parameter expansion. This simple script can be modified further based on your needs and can serve as a building block for more complex string manipulations in your Bash scripts.
FAQ
-
Can I modify the script for different strings?
Absolutely! You can replace the content of the
string
variable with any other string you wish to process. -
What if my string is empty?
If the string is empty, the modified string will also be empty, and no error will occur.
-
Is this method compatible with all versions of Bash?
Yes, the parameter expansion feature used here is supported by modern Bash versions.
-
How can I remove multiple characters from the end?
You can adjust the parameter expansion to remove multiple characters by using
string::-(n)
, wheren
is the number of characters you want to remove. -
Can I use this script inside a larger Bash script?
Yes, you can include this logic in larger scripts where you need to manipulate strings.
Troubleshooting
Here are some common issues you might encounter with this script:
- Permission Denied Error: This occurs if you haven’t given the script execution permissions. Use
chmod +x script_name.sh
. - Command Not Found: Ensure you are in the correct directory and that you are calling the script with the proper name.
- Script Not Modifying the String: Check that the variable names are correctly referenced and that you’ve properly implemented parameter expansion.