In the world of programming and scripting, text manipulation is a fundamental task. A common requirement is to convert a given string or sentence to uppercase. This article will guide you through creating a simple Bash script that accepts inputs via the command line interface (CLI) and transforms them into uppercase letters.
Prerequisites
- A basic understanding of Bash scripting
- Familiarity with command line operations
- Access to a Unix-based system (Linux or macOS)
DID YOU KNOW?
The first letter case conversion utility was introduced in UNIX systems in the 1970s. Since then, it has been a standard feature in most programming languages.
The Script
Below is a simple Bash script that takes a string or sentence as an argument and converts it to uppercase. The script utilizes the built-in tr
command, which translates characters from one set to another.
#!/bin/bash
if [ $# -eq 0 ]; then
echo "Usage: $0 string"
exit 1
fi
echo "$1" | tr '[:lower:]' '[:upper:]'
Step-by-Step Explanation
NOTE!
It’s crucial to ensure that you pass a string as an argument; otherwise, the script will prompt for usage.
This script is straightforward but effective. Let’s break down each part to understand its functionality.
- Shebang Line: The first line
#!/bin/bash
indicates to the system that this script should be run in the Bash shell. - Argument Check: The line
if [ $# -eq 0 ]; then
checks if any arguments were passed to the script. If none were provided, it displays usage instructions. - Uppercase Conversion: The command
echo "$1" | tr '[:lower:]' '[:upper:]'
takes the first argument (the string) and pipes it to thetr
command which converts all lowercase characters to uppercase.
How to Run the Script
To execute the script, follow these simple steps:
- Open your terminal.
- Make the script executable with the command:
chmod +x script_name.sh
. - Run the script by providing a string as an argument:
./script_name.sh "your text here"
.
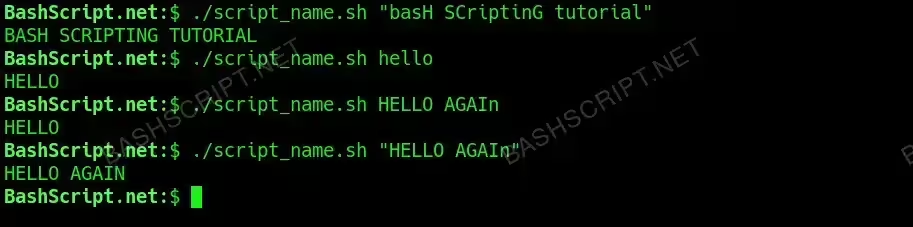
Conclusion
In this article, you learned how to create a Bash script that converts strings to uppercase. This simple yet powerful utility can be enhanced further to handle multiple words or sentences by extending the script’s logic. Bash scripting offers significant capabilities for text processing and automation.
FAQ
-
Can I modify the script to convert to lowercase?
Yes, you can replace
tr '[:lower:]' '[:upper:]'
withtr '[:upper:]' '[:lower:]'
for converting text to lowercase. -
What if the script doesn’t execute?
Ensure that you have the necessary execute permissions and that the script has been saved correctly.
-
Is it possible to convert multiple words at once?
Yes, by modifying the script to handle
"$@"
instead of"$1"
, you can process multiple arguments in a single execution. -
Can I run this script on Windows?
You typically need to use a Unix-like environment, such as WSL (Windows Subsystem for Linux), to run Bash scripts on Windows.
-
What if I forget to provide an argument?
The script includes a check that ensures usage instructions are displayed if no argument is provided.
Troubleshooting
Here are some common errors and solutions related to this script:
- Error:
command not found
when running the script. Solution: Make sure the script has executable permissions set usingchmod
. - Error: Improper usage message is displayed. Solution: Ensure that you are passing a string as an argument when executing the script.
- Error: Unexpected output. Solution: Check if any special characters in the input are affecting the output.