In the world of digital logic, XOR gates play a crucial role in determining outputs based on binary inputs. When translated into a Bash script, implementing an XOR operation can facilitate decision-making processes and enhance various programming tasks. This article will guide you through the practical usage of XOR gates in a Bash script, helping you understand how to effectively implement logic operations in shell scripting.
Prerequisites
- Basic understanding of Bash scripting.
- Familiarity with
if
statements andconditional expressions
. - Knowledge of logical operators in Bash.
- No external packages are required; a standard Bash installation suffices.
DID YOU KNOW?
An XOR (exclusive OR) gate outputs true only when exactly one of its inputs is true. This property makes it valuable for various applications in computer science and digital systems.
The Script
The following Bash script demonstrates how to implement an XOR operation between two binary inputs. The script checks the values provided and computes the result based on the XOR logic.
#!/bin/bash
# Function to perform XOR operation
xor() {
if [ "$1" -ne "$2" ]; then
echo "1"
else
echo "0"
fi
}
# Main script execution
input1=$1
input2=$2
echo "XOR Result: $(xor $input1 $input2)"
Step-by-Step Explanation
NOTE!
Ensure that the inputs provided to the script are either 0 or 1 for correct functionality.
In this section, we will break down the provided script to understand each part clearly.
- Define the XOR Function: The
xor
function takes two arguments and compares them using theif
statement to check if they are different. - Accept User Input: The script retrieves the first and second inputs from command line arguments using positional parameters
$1
and$2
. - Execute XOR Operation: The script calls the
xor
function with the provided inputs and outputs the result. - Output the Result: Finally, the script prints the result of the XOR operation.
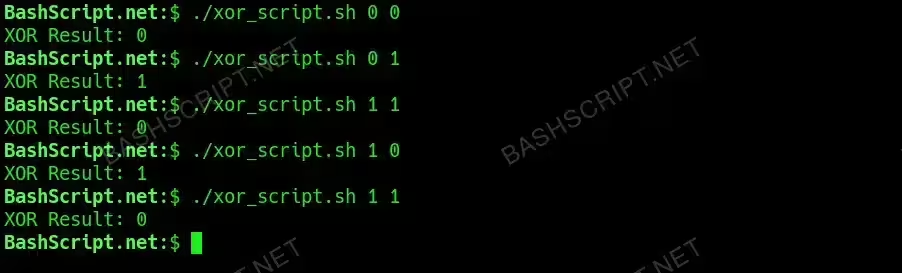
How to Run the Script
To execute the script, follow these straightforward steps:
- Open your terminal.
- Save the script to a file (e.g.,
xor_script.sh
) and give it executable permissions using the commandchmod +x xor_script.sh
. - Run the script with two binary inputs:
./xor_script.sh 1 0
or./xor_script.sh 0 0
.
Conclusion
Using XOR gates in Bash scripting can significantly enhance the way logic operations are handled in digital problem-solving. By understanding how to implement such operations, you can create more complex scripts that leverage binary logic effectively. This knowledge can be applied in various programming scenarios, making your scripts smarter and more versatile.
FAQ
-
What happens if I provide non-binary inputs?
The script assumes only 0 or 1 as valid inputs. Non-binary inputs may lead to unexpected results.
-
Can I modify the script for more inputs?
Yes, but you will need to adjust the logic to handle multiple comparisons.
-
Is the XOR operation commutative?
Yes, XOR is commutative, meaning the order of the inputs does not affect the result.
-
Can this script be used in larger projects?
Absolutely! This basic XOR implementation can serve as a building block for larger logical operations in complex scripts.
Troubleshooting
Here are some common issues you may encounter while running the XOR script:
- Permission Denied: Ensure the script is executable by running
chmod +x xor_script.sh
. - Invalid Input: Check that you are only passing 0 or 1 as inputs.
- Command Not Found: Ensure you are in the directory where the script is located or provide the correct path.