When it comes to managing variables in Bash, appending values to an existing variable is a common task. This article will provide a straightforward example of how to append to a variable within a Bash script, which can be extremely useful for various scripting scenarios.
Prerequisites
- Basic understanding of Bash scripting
- Familiarity with variables in Bash
- Knowledge of arrays (optional, but helpful)
- Experience with
echo
andread
commands
DID YOU KNOW?
Appending to a variable can help you build strings dynamically, making your scripts more flexible and powerful.
The Script
Here’s a simple script that demonstrates how to append to a variable in Bash. In this example, we’ll take inputs from the user and append the input values to a single variable.
#!/bin/bash
# Initialize an empty variable
my_var=""
# Read multiple inputs and append them
while true; do
read -p "Enter a value (or type 'exit' to finish): " input
if [ "$input" == "exit" ]; then
break
fi
my_var+="$input "
done
# Display the final result
echo "You've entered: $my_var"
Step-by-Step Explanation
NOTE!
This script continuously prompts the user for input until they type ‘exit’.
In this section, we will explain how the script works step-by-step.
- Initialization: We start by initializing an empty variable called
my_var
. - User Input: The script prompts the user for a value using
read
. - Condition Check: If the user types ‘exit’, the while loop terminates.
- Appending Values: If a valid input is provided, the input is appended to
my_var
. - Display Result: Once finished, the script displays the complete value of
my_var
.
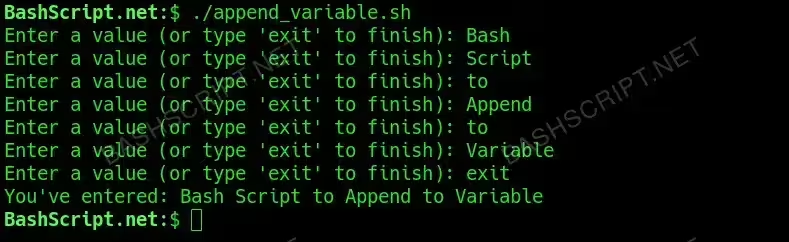
How to Run the Script
To execute the script, follow these steps:
- Create a new file, for example
append_variable.sh
. - Copy the provided script code into your file.
- Make the script executable by running
chmod +x append_variable.sh
. - Run the script with
./append_variable.sh
.
Conclusion
Appending to a variable in Bash is a simple yet powerful technique that can help streamline your scripts. With the above example, you can easily gather user input and build a cohesive string dynamically to fit your needs.
FAQ
-
Can I append to a variable in a function?
Yes, you can append to a variable defined outside a function, or you can define local variables within the function scope.
-
What will happen if I forget to initialize my variable?
If you try to append to an uninitialized variable, it will be treated as an empty string, and the appended values will still work.
-
Can I append output from other commands?
Absolutely! You can append the output of commands by using command substitution, e.g.,
my_var+="$(command)"
. -
What if I want to append without extra spaces?
You can append without spaces by using different syntax, e.g.,
my_var+="$input"
without the space.
Troubleshooting
Here are some common errors you might encounter while appending to variables and how to resolve them:
- Unexpected token error: This usually happens if there’s a syntax error in the script. Double-check for missing quotes or semicolons.
- Variable not displaying as expected: Ensure you used
echo
correctly and are evaluating the variable at the right point in the script. - Infinite loop: If you forget the exit condition, the script will keep prompting indefinitely. Always implement a way to exit loops.