Bash scripting is a powerful way to automate tasks in Unix-like operating systems. One of the most common tasks is redirecting output to a file. In this article, we will provide a comprehensive example of a Bash script that outputs data to a file, along with explanations and instructions on how to use it effectively.
Prerequisites
DID YOU KNOW?
You can output the results of any command directly to a file using the >
operator in Bash.
The Script
This script generates a list of active users and their current shell sessions, redirecting the output to a file named active_users.txt
. It utilizes the who
command to gather the necessary information.
#!/bin/bash
# This script outputs active user sessions to a file
who > active_users.txt
echo "Active users have been saved to active_users.txt"
Step-by-Step Explanation
NOTE!
Ensure you have permission to write to the directory where you plan to run this script.
Let’s break down the script step by step:
- Shebang Line: The first line
#!/bin/bash
specifies the script interpreter to be used. - Output Command: The
who
command retrieves information about users currently logged into the system. - Redirect to File: Using
>
symbol redirects the command output toactive_users.txt
. - Echo Feedback: The final line provides feedback confirming that the information has been saved.
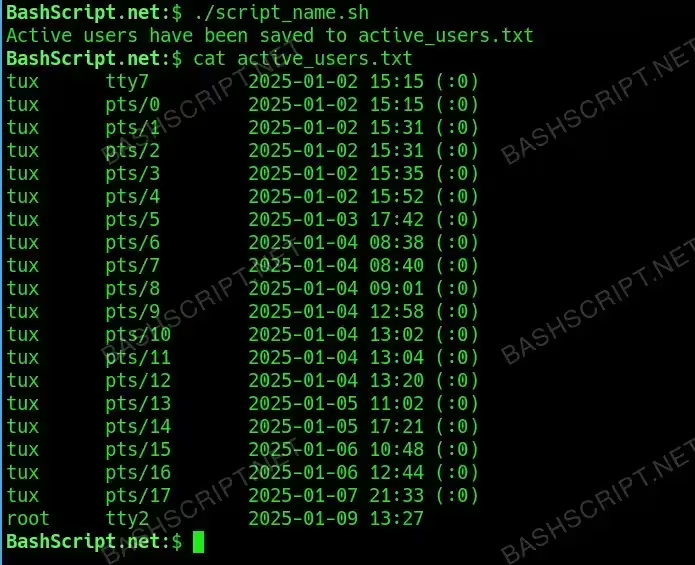
How to Run the Script
To execute the script, follow these simple steps:
- Open your terminal.
- Navigate to the directory where your script is saved. For example:
cd /path/to/script
. - Run the script using the command:
bash script_name.sh
, replacingscript_name.sh
with the actual script file name.
Conclusion
Outputting to a file in Bash is a fundamental skill that can enhance your scripting capabilities. This script example illustrates how easy it is to capture user information and redirect output, paving the way for further automation and data collection tasks.
FAQ
-
How do I check the content of the output file?
Use the command
cat active_users.txt
to display the contents of the file in the terminal. -
What should I do if the script has permission issues?
Make sure to grant execute permissions using
chmod +x script_name.sh
. -
Can I modify the output file name?
Yes, simply change
active_users.txt
to the desired file name in the script. -
How can I run this script automatically at intervals?
Consider using cron jobs to schedule the script execution at regular intervals.
-
Is there any way to append to the output file rather than overwrite it?
Yes, use
>>
instead of>
in the script.
Troubleshooting
Here are some common errors related to this script and how to resolve them:
- Permission Denied: You may be trying to write to a read-only directory. Resolve this by running the script in a writable directory.
- No such file or directory: Ensure you are running the script from the correct location. Verify your current directory using
pwd
. - Command not found: Ensure that you have Bash installed and you’re running the script in a Bash environment.