Bash scripting is an essential skill for anyone looking to automate tasks in a Linux environment. One of the powerful features of Bash scripts is the use of positional arguments, which allows you to pass data directly to your scripts from the command line. This article will guide you through the basics of using positional arguments, specifically focusing on how to utilize variables like $1
, $2
, and beyond, to enhance your scripting capabilities.
Prerequisites
- A basic understanding of Bash scripting
- Familiarity with variables, functions, and conditional statements
- Access to a Linux terminal with Bash installed
DID YOU KNOW?
You can pass up to 9 positional parameters to your Bash script, using $1
to $9
. If you need more, you can use ${10}
, ${11}
, etc.
The Script
This simple Bash script demonstrates how to utilize positional arguments. The script takes two arguments and performs a basic operation, such as adding two numbers together.
#!/bin/bash
# A simple script to add two numbers passed as arguments
if [ $# -ne 2 ]; then
echo "Usage: $0 "
exit 1
fi
sum=$(($1 + $2))
echo "The sum of $1 and $2 is $sum."
Step-by-Step Explanation
NOTE!
Ensure that you provide exactly two numeric arguments when running the script to avoid errors.
Let’s break down the script step by step:
- Shebang Line: The line
#!/bin/bash
tells the system to execute the script using Bash. - Argument Check: The
if [ $# -ne 2 ]; then
condition checks if the number of arguments provided is not equal to 2. If this condition is true, the usage message is displayed and the script exits. - Perform Addition: The expression
sum=$(($1 + $2))
takes the first and second arguments and calculates their sum. - Output Result: Finally, the result is printed using
echo
.
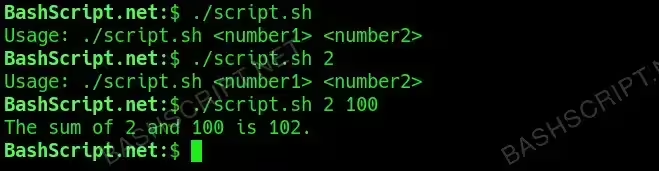
How to Run the Script
Running the script is straightforward. Follow these steps:
- Open your terminal.
- Navigate to the directory where your script is located using
cd /path/to/directory
. - Make the script executable by running
chmod +x script_name.sh
(replacescript_name.sh
with your actual script name). - Execute the script with two numbers as arguments:
./script_name.sh 5 3
.
Conclusion
Understanding positional arguments in Bash scripts can significantly improve your scripting efficiency by allowing for flexible inputs. With this foundational knowledge, you can begin to leverage arguments in more complex scripts, enhancing automation tasks and interactivity in your scripts.
FAQ
-
What if I provide more than two arguments?
The script currently only accepts two arguments and will display a usage message if more or fewer arguments are provided.
-
Can I pass arguments that are not numbers?
Yes, but the script will attempt to perform arithmetic on them, which could lead to unexpected results or errors.
-
How can I access more than nine positional arguments?
You can access them using the syntax
${10}
,${11}
, and so on. -
What does the
$#
variable represent?The
$#
variable holds the number of positional parameters passed to the script.
Troubleshooting
Here are some common error messages you might encounter while using positional arguments in Bash scripts and how to resolve them:
- Error: “Usage: script_name.sh “ – This means you didn’t provide the correct number of arguments. Check your input.
- Error: “expression requires a context” or “invalid arithmetic operator” – Ensure you are passing numeric values. Non-numeric inputs will cause these errors.