In today’s interconnected world, knowing the active devices on your network can be essential for various administrative tasks and security measures. One efficient way to scan for alive hosts is by using a Bash script that pings every IP address within a specified subnet. This article will guide you through writing such a script, starting from the prerequisites to executing it on your machine.
Prerequisites
- Basic knowledge of Bash scripting including variables, loops, and functions.
- Command-line interface access (such as Terminal in Linux or macOS, or Command Prompt/PowerShell in Windows with WSL).
- The ping utility installed on your system (this usually comes pre-installed on most Linux distributions and macOS).
DID YOU KNOW?
Scanning your network regularly can help identify unauthorized devices, which is crucial for maintaining network security.
The Script
This script scans a given subnet to identify active hosts by sending a ping to each IP address within the range specified. The user provides the subnet in CIDR notation, which the script uses to iterate through and check which addresses respond.
#!/bin/bash
if [ -z "$1" ]; then
echo "Usage: $0 <CIDR>"
exit 1
fi
IFS='/' read -r base_ip range <<< "$1"
IFS='.' read -r i1 i2 i3 i4 <<< "$base_ip"
total_ips=$((2 ** (32 - range) - 2))
for ((ip=1; ip<=total_ips; ip++)); do
current_ip="$i1.$i2.$i3.$((i4 + ip))"
ping -c 1 -W 1 "$current_ip" &> /dev/null && echo "$current_ip is alive" &
done
wait
echo "Scan complete!"
Step-by-Step Explanation
NOTE!
Ensure you have permission to scan the network, as unauthorized scans can lead to security alarms.
Let’s break down the script step-by-step to understand how it functions:
- Check for User Input: The script begins by checking if the user has provided a CIDR notation as an argument.
- Parse the CIDR: Using the internal field separator (IFS), the script splits the input to get the base IP and range bitmask.
- Calculate Active IPs: It calculates the total number of usable IPs by determining the range size and excluding network and broadcast addresses.
- Ping Each Address: The script iterates through all possible IP addresses, pings each one, and prints the status of alive hosts in parallel for efficiency.
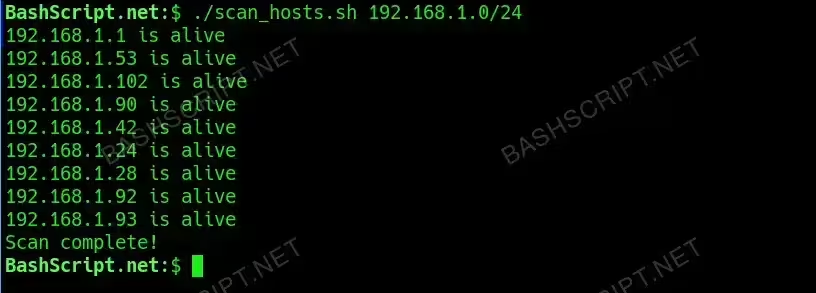
How to Run the Script
Running the script is straightforward. Just follow these steps:
- Open your terminal window.
- Copy the script into a file and save it as
scan_hosts.sh
. - Make the script executable with the command:
chmod +x scan_hosts.sh
. - Run the script providing a CIDR range:
./scan_hosts.sh 192.168.1.0/24
.
Conclusion
This Bash script provides an efficient way to find alive hosts on your network. By setting it up and running it with the correct CIDR notation, you can quickly identify active devices, which can help in network management and security efforts.
FAQ
-
What is a CIDR notation?
CIDR notation is a compact representation of an IP address and its associated network mask, allowing for efficient allocation of IPs.
-
Can I run this script on Windows?
Yes, you can run it using Windows Subsystem for Linux (WSL) or Git Bash.
-
What if the script doesn’t run?
Ensure you have executable permissions on the script file and that Bash is installed on your operating system.
-
Will this script affect my network performance?
While the script runs multiple pings in parallel, it’s a good practice to run it during non-peak hours to avoid potential network congestion.
-
What happens if an IP is not reachable?
If an IP address does not respond to the ping, it will simply not be printed as alive.
Troubleshooting
If you encounter issues while running the script, here are some common error messages and their solutions:
- “Usage: ./scan_hosts.sh ”: This indicates you did not supply a CIDR address. Make sure to input a valid subnet.
- “Ping request could not find host X.X.X.X”: This may occur if the IP address is invalid or not assigned to a device on the network.
- “Permission denied”: Check if the script has the appropriate executable permissions with
chmod +x scan_hosts.sh
.