In the world of automation and scripting, Bash scripts are indispensable tools for processing data. This article aims to guide you through creating a Bash script that efficiently accepts input from a pipe, reads the data line-by-line, and processes it effectively. Whether you are looking to automate repetitive tasks or handle piped data seamlessly, this script can be an essential addition to your toolkit.
Prerequisites
DID YOU KNOW?
Almost all Unix-like operating systems support piping, which allows you to connect multiple commands in sequence, passing output from one command to the next!
The Script
This script will read input data from the standard input (stdin), process each line, and perform a specific action on the data. Below is a simple script example that trims whitespace from the start and end of each line:
#!/bin/bash
while IFS= read -r line; do
echo Piped data: "${line}" | xargs
done
Step-by-Step Explanation
NOTE!
This script uses the read
command to read input line-by-line and xargs
to trim off any excess whitespace.
The following steps break down how the script works and what each component does:
- Shebang: The script starts with
#!/bin/bash
, indicating that it should run in the Bash shell. - Reading Input: The line
while IFS= read -r line; do
sets up a loop to read from standard input line-by-line. - Processing Each Line: Inside the loop,
echo "${line}" | xargs
is used to remove any leading or trailing whitespace from each line. - Ending the Loop: The loop ends with
done
, which signifies that all input has been processed.
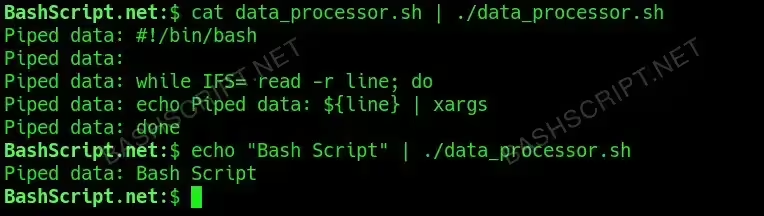
How to Run the Script
To execute the script and see it in action, follow these simple steps:
- Open a terminal window.
- Create a new Bash script file, for example,
data_processor.sh
, and paste the script into it. - Make the script executable using the command
chmod +x data_processor.sh
. - Run the script with piped input, for example:
cat input.txt | ./data_processor.sh
.
Conclusion
Creating a Bash script that accepts input from a pipe is straightforward yet powerful for automating tasks and processing data. This article has outlined the foundational steps and provided a basic script to get you started on your scripting journey.
FAQ
-
What does the xargs command do?
The
xargs
command is used to build and execute command lines from standard input, which in this case helps to remove whitespace from strings. -
Can I modify the script for different types of data?
Yes! You can replace the
echo "${line}" | xargs
part with any command you want to use for processing each line of input. -
How do I debug the script?
You can add
set -x
at the beginning of the script to enable debugging output, which shows each command being executed. -
Is this script portable?
The script should work on any system with a Bash shell and the necessary permissions to run scripts.
-
What do I do if the script doesn’t run?
Ensure that you have made the script executable and that you are providing the correct input through piping.
Troubleshooting
Here are some common error messages you might encounter while running the script, along with their solutions:
- Permission denied: Make the script executable with
chmod +x script_name.sh
. - Command not found: Ensure you are running the script from a Bash shell and have correctly typed the script name.
- Input is empty: Check that the input data is being piped correctly from the previous command.