Flags in Bash scripts are crucial for modifying the behavior of scripts without needing to pass lengthy arguments. Particularly, flags without arguments provide a simple way to enable or disable features in your scripts, often making your scripts more user-friendly and easier to use. This article covers how to effectively use and parse these types of flags in your Bash scripts.
Prerequisites
- Basic understanding of Bash scripting
- Familiarity with conditional statements
- Knowledge of functions and variables
- Awareness of the getopts built-in command
DID YOU KNOW?
In Bash scripting, flags can be single-letter options or longer multi-letter options, and using them can significantly simplify user input handling.
The Script
This script demonstrates a basic Bash implementation that utilizes flags without arguments to control features such as enabling debug mode or verbose output.
#!/bin/bash
verbose=false
debug=false
while getopts "vd" flag; do
case "$flag" in
v) verbose=true ;;
d) debug=true ;;
*) echo "Usage: $0 [-v] [-d]"
exit 1 ;;
esac
done
if $verbose; then
echo "Verbose mode enabled."
fi
if $debug; then
echo "Debugging mode enabled."
fi
echo "Script running..."
Step-by-Step Explanation
NOTE!
The getopts
command is essential for parsing flags in this script. It helps streamline the process of checking for options.
This script allows the user to toggle verbose and debugging modes using flags. Here’s how it works:
- Define Default Values: Initially set
verbose
anddebug
tofalse
. - Parse Flags: Use
getopts
to parse the flags. Each flag corresponds to a case in the switch statement. - Check Flags: After parsing, check the status of
verbose
anddebug
, and respond accordingly. - Execute Script: Finally, proceed with running the script based on the enabled flags.
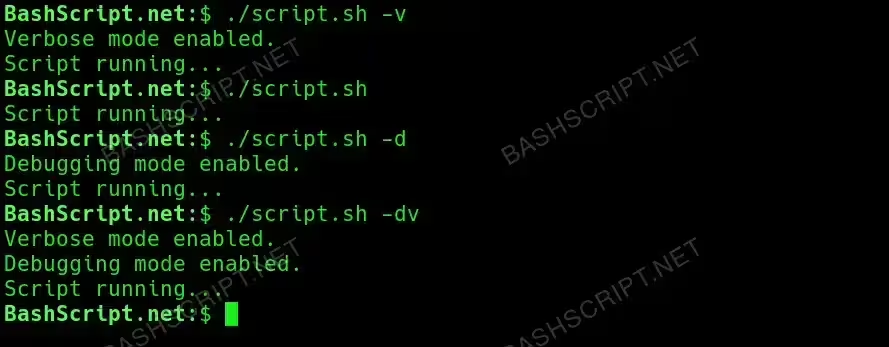
How to Run the Script
To execute the script, follow these steps:
- Open your terminal.
- Navigate to the directory containing the script.
- Run the script with the desired flags, e.g.,
./script.sh -v -d
.
Conclusion
Using flags without arguments in Bash scripts helps streamline user interactions, allowing for simpler input while keeping the script’s functionality intact. By employing getopts
, you can effectively manage options and improve the overall usability of your scripts.
FAQ
-
What happens if I use an invalid flag?
The script will display the usage message and exit. You can handle this in the
default
case of the switch statement in yourgetopts
options. -
Can I add more flags?
Yes, you can easily extend the
getopts
string and add more cases in the switch statement for additional flags. -
Is it possible to combine flags?
Absolutely! Combine flags like this:
-vd
to enable both verbose and debug modes simultaneously. -
What is the difference between flags with and without arguments?
Flags without arguments simply toggle features on or off, while flags with arguments require an additional value to specify the option’s behavior.
Troubleshooting
Here are some common issues and solutions related to flag parsing in Bash scripts:
- Error: Invalid option specified: Ensure you are using only the defined flags.
- Error: Script not executing: Ensure you have made the script executable with
chmod +x script.sh
. - Unexpected behavior: Double-check your flag parsing logic and ensure all flags are accounted for in the case statements.