In this article, we’ll explore how to create a simple Bash script that uses SSH to connect to a remote host with a username and password. Though using a password in scripts is generally discouraged due to security reasons, this example aims to demonstrate the mechanics of SSH connections in Bash scripting.
Prerequisites
- A basic understanding of Bash scripting.
- Familiarity with SSH and how it works.
- Access to a remote server for testing purposes.
- Ensure required packages like sshpass are installed on your system.
DID YOU KNOW?
You can use SSH keys for authentication, which is considered more secure than using passwords in scripts!
The Script
This script demonstrates how to establish an SSH connection by hardcoding your username, password, and host. Please note that this method is less secure and should only be used for demonstration or in controlled environments.
#!/bin/bash
# Hardcoded variables
HOST="your.remote.server"
USER="your_username"
PASSWORD="your_password"
# Using sshpass for SSH login
sshpass -p "$PASSWORD" ssh "$USER@$HOST"
Step-by-Step Explanation
NOTE!
Always be cautious with hardcoding sensitive information in your scripts.
Let’s break down the script sections so you can understand how it works:
- Define Variables: The script starts with defining variables for the host, user, and password. Feel free to replace the placeholder texts with actual values.
- Invoke SSH with sshpass: By using
sshpass
, we can pass the password to the SSH command without manual input. - Connect to the Host: The script will connect to the specified
HOST
using the providedUSER
andPASSWORD
.
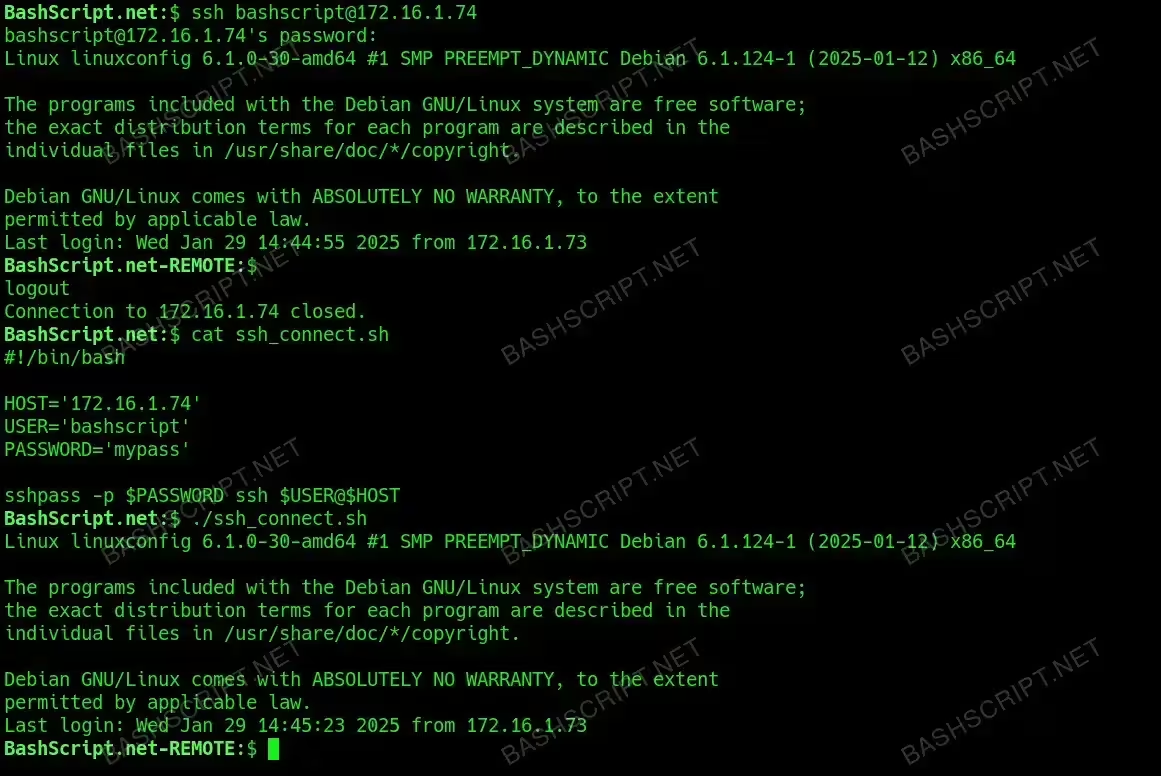
How to Run the Script
Once you have saved the script as, for instance, ssh_connect.sh
, follow these steps to execute it:
- Open your terminal.
- Navigate to the directory where your script is saved.
- Run the script with the command
./ssh_connect.sh
.
Conclusion
This script is a straightforward example of connecting to a remote server via SSH using hardcoded credentials. Remember, while this can be useful for quick testing, it is highly recommended to use SSH keys for secure authentication in production environments.
FAQ
-
What is sshpass?
sshpass is a utility that allows you to provide a password in a command line interface for SSH connections.
-
Is it safe to use hardcoded passwords in scripts?
No, hardcoding passwords can expose your credentials. It’s better to use SSH keys or other secure methods.
-
What if sshpass is not installed?
You can install it using your package manager. For example, on Debian-based systems, use
sudo apt-get install sshpass
. -
Can I use this script with multiple hosts?
Yes, you can modify the script to loop over an array of hosts and perform actions on each one.
Troubleshooting
If you encounter issues, consider the following common errors:
- Permission Denied: Check if your username and password are correct and if you have access to the host.
- sshpass: command not found: Ensure that
sshpass
is installed and accessible in your system’s PATH. - Connection timed out: Verify that the host is reachable from your network and that SSH services are running on the host.